In previous post, I’ve talked about compiling TOR from source in Android and added some helper libraries for starting and configuring TOR. In this post, I’ve created a library based on Tor Binary (version 0.4.4.0) and published in GitHub, JFrog and JitPack.
Process
Whole Process is simple. The following picture depicts it.

Importing The TOR Android into Android Studio
There are 3 ways for importing the AAR library to Android Studio.
- Download the latest release from TOR Android Github Page.
- Import from jCenter
implementation 'ir.mstajbakhsh:tor-android:1.0.0'
- Get from JitPack
allprojects { repositories { maven { url 'https://jitpack.io' } } } dependencies { implementation 'com.github.mirsamantajbakhsh:TorAndroid:1.0.0' }
Sample Code
Here is a sample code for starting the TOR Android, an application with only one activity. The only needed permission is INTERNET which should be added to the Manifest.
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); AsyncTask.execute(new Runnable() { @Override public void run() { final TorProxy tb = new TorProxy.TorBuilder(getApplicationContext()) .setSOCKsPort(TOR_TRANSPROXY_PORT_DEFAULT) //9150 for example .setUseBrideges(false) .setExternalHiddenServicePort(80) .setInternalHiddenServicePort(80) .setDebuggable(false) .build(); tb.init(); runOnUiThread(new Runnable() { @Override public void run() { Toast.makeText(getApplicationContext(), "Initialized", Toast.LENGTH_LONG).show(); } }); try { tb.start(new IConnectionDone() { @Override public void onSuccess() { Log.d("TorAndroid", "Tor started successfully."); runOnUiThread(new Runnable() { @Override public void run() { Toast.makeText(getApplicationContext(), "TOR is OK.", Toast.LENGTH_LONG).show(); Toast.makeText(getApplicationContext(), tb.getOnionAddress(), Toast.LENGTH_LONG).show(); } }); } @Override public void onFailure(final Exception ex) { Log.e("TorAndroid", "Error in starting Tor.\r\n" + ex.getMessage()); runOnUiThread(new Runnable() { @Override public void run() { Toast.makeText(getApplicationContext(), "Error\r\n" + ex.getMessage(), Toast.LENGTH_LONG).show(); } }); } }); } catch (IOException e) { e.printStackTrace(); } } }); }
The result is OK. See it here:
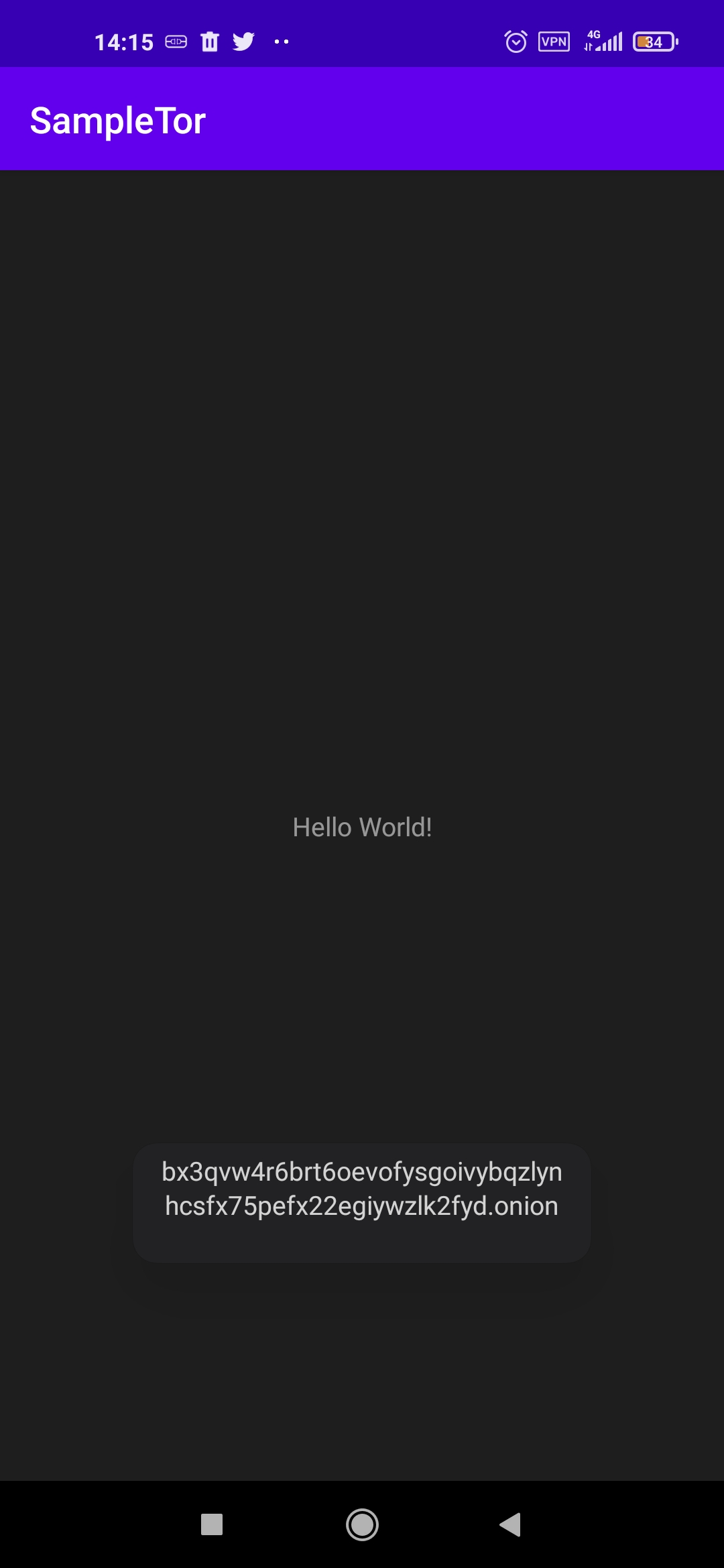